Anatomy of a Webpack Loader
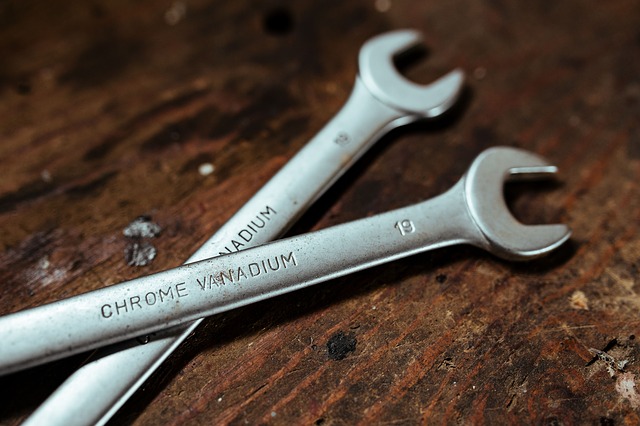
(source)
This is my attempt at an extremely abridged version of explaining what a Webpack Loader does 😅
aka. Webpack loader basics
Q: Why do we use Webpack Loaders?
Webpack Loaders help to transform non-JavaScript imports into useable JavaScript values.
Examples:
css-loader
: Exposes.css
styling information as a JS objectyaml-loader
: Exposes.yaml
file contents as a JS objectfile-loader
: Emits file in build + exposes file as static URL
Loader: Basic Structure
A webpack loader principally does 3 things:
-
Read contents of a file
- Loaders are used in Webpack Rules (see more)
- Each rule contains options to match/exclude files (eg.
Rule.test
,Rule.include
,Rule.exclude
, etc…) - Matched files will be fed into the specified loader
- When files are fed into a loader, the loader will have access to the contents of each file
See more: Example Webpack Rule definition
// webpack.config.js module.exports = { module: { rules: [ // other rules... { test: /locales\.json$/, type: "javascript/auto", loader: require.resolve("i18n-locales-loader"), options: { esModule: true, buildLocalesPath: ["static", "locales"], }, }, // other rules... ], }, };
-
Export JavaScript variables
- It’s usually useful to expose some JavaScript information based on the input file content.
- Eg.
yaml-loader
# someYamlFile.yaml --- name: samuel
- Given
import yamlContent from './someYamlFile.yaml'
+yaml-loader
, - In order for
yamlContent
to contain the information from the YAML file (ie.{"name": "samuel"}
), yaml-loader
reads the YAML file (see loader code),yaml-loader
exports the YAML file contents usingexport default
(see loader code)
-
Emit files
- Depending on the use case, a loader can choose to emit file(s). (ie. not all loaders emit files.)
- Eg.
file-loader
- Given
import svgFile from './someSvgFile.svg'
+file-loader
, - In order for
svgFile
to contain a string referring to the static asset’s URL, file-loader
emits the.svg
file (see loader code),file-loader
constructs the static asset’s URL (see loader code),file-loader
exports the URL as a string usingexport default
(see loader code)
-
(Bonus) Do anything else
- Technically, since a loader is written in JavaScript, a loader can contain other code for telemetry or build statistics purposes.
Explained Example: i18n-locales-loader
Note: Yes, this is a shameless plug of my own i18n-locales-loader
webpack loader 😂
Summary: i18n-locales-loader
(GitHub Repo) splits up locales.json
files into locale-specific files eg. en.json
, id.json
What it does:
- Parses
locales.json
to a JSON object (see loader code) - Creates JSON object for every locale (see loader code)
- Generates static asset URL path for every locale (see loader code)
- Emits
.json
file for every locale (see loader code) - Creates JSON object containing locale to static asset URL path mapping (see loader code)
- Exports JSON object using
export default
(see loader code)
Summary and References
- For purposes of education, I have used simpler loaders to more clearly illustrate the key principles of a webpack loader.
- For an example of the full potential of a loader, you may take a look at
vue-loader
and an explanation of how it works. (Warning: It’s very complex! 🤯) - Other references:
- SurviveJS: Webpack: Good resource for a more rigorous treatment of explaining what Webpack does.
- Loaders: Webpack: Official Webpack documentation for Loaders
- Concepts: Webpack: Official Webpack documentation
I hope this article helps with understanding how webpack loaders work, and perhaps even help you in crafting your own loaders! 🙃